ETEE Examples
Filters
Below are some examples of using the Filter command
available with ETEE. These examples use Unix commands such
as tr, perl, sed,
uuencode, uudecode, and wc.
Windows users can install these commands on their systems
using Cygwin or perhaps finding other
Windows binaries.
The command
tr '[a-z]' '[A-Z]'
will translate
lowercase to uppercase. Conversely, the command
tr '[A-Z]' '[a-z]'
will translate
uppercase to lowercase.Using Perl, you can write utilities to generate content, such as HTML table generation. Define a Perl script mktbl.pl (in a directory in your PATH) as follows :
# mktbl.pl - make an HTML table.
# Usage: mktbl nrows ncols
my ($rows, $cols) = @ARGV;
$rows = 3 if (! defined $rows );
$cols = 4 if (! defined $cols );
print("<table>\n");
for ($row = 0; $row <= $rows; $row++)
{ printRow($row, $cols, "th", $row == 0 ? "column" : "data $row,") }
print("</table>\n");
sub printRow() {
my ($row, $num, $elt, $label) = @_;
print(" <tr> <!-- row $row -->\n");
for ($col = 1; $col <= $num; $col++) {
print(" <$elt>$label $col</$elt>\n");
}
print(" </tr>\n");
} # end mktbl.pl
mktbl.pl 6 3
to make an HTML table with 6 rows and 3 colums. If you
are editing HTML within a Javadoc comment, extend the command
into a pipeline as follows:
mktbl.pl 6 3 | sed "s/^/ * /"
This will generate the table and prefix each line with
" * " as part of the comment.Eclipse can tell you how many lines are in the current file, but not how many lines or characters or words are in the selected region. Select the region, then invoke the filter
wc
Note: Invoking
wc will replace the region with the
output of the filter, but after seeing the output, you can
easily Undo the change.To count just the number of lines in a long method of a Java class, select the method body and run the filter
wc -l
(Note: a possible enhancement for ETEE would be an
option to run a filter but direct the output to the
Eclipse console log window instead of replacing the selected
region.)Quoting and Unquoting XML Special Characters
Say you wish to add the following text to an HTML document, or inside a Java source file as javadoc. The text is not valid HTML - there is an open < which does not begin a HTML element, and there is an open & which does not begin a valid HTML entity.
< is replaced with <
> is replaced with >
& replaced with &
" replaced with "
You wish to sort this text first, then convert it to
valid HTML. TO sort the text, select all the lines of text
and invoke Filters -> Filter and enter sort as the
command. (This assumes you have a valid sort command in your
PATH, of course.)> is replaced with >
& replaced with &
" replaced with "
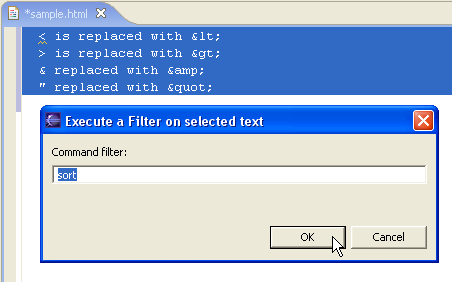
After you click OK, the sorted text replaced the selected region:
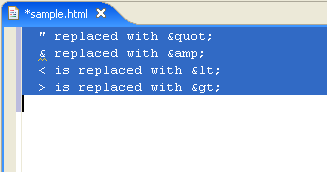
Now, we have to convert the text to valid HTML. This means changing each & to & and changing each < to <, etc. While the region is still selected, choose the Quote XML Special Characters menu item:
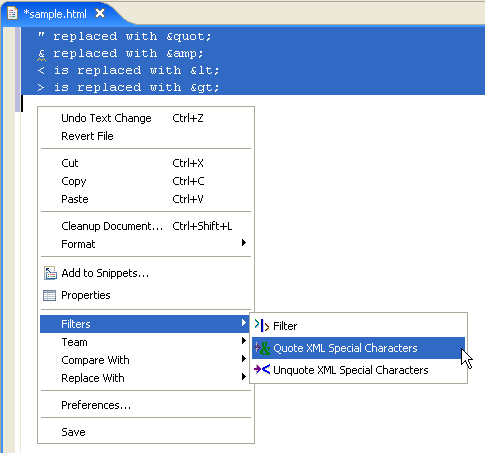
" replaced with &quot;
& replaced with &amp;
< is replaced with &lt;
> is replaced with &gt;
This quoted XML is a bit harder to read and to work
with. You can use the Unquote XML Special Characters action
to do the reverse: convert valid XML back into raw text. This
makes it easier to edit the text. When you are done editing
it as text, reconvert the special characters back to
XML.& replaced with &amp;
< is replaced with &lt;
> is replaced with &gt;